How to easily install requests with pip and python
The requests library is the de facto standard for making HTTP requests in Python. It abstracts the complexities of making requests behind a beautiful, simple API so that you can focus on interacting with services and consuming data in your application.
Some use cases for requests include Web Scraping, API integration, data retrieval from web services, automated testing of web applications, and sending data to remote servers.
In this tutorial, we’ll cover several ways to install requests and demonstrate basic usage with some examples.
Installing requests
There are several ways to install requests depending on your needs and environment.
Using pip
The easiest way to install requests is using pip:
python -m pip install requests
Using conda
If you use the Anaconda distribution of Python, you can install requests using conda:
conda install requests
Using a virtual environment
It’s good practice to use a virtual environment to manage the dependencies for your Python projects. You can install requests in a virtual environment like so:
python -m venv myenv
source myenv/bin/activate # On Windows, use `myenv\Scripts\activate`
pip install requests
From source
You can also install requests from source by downloading the code from the GitHub repo and running:
python setup.py install
Troubleshooting
If you encounter issues when trying to install requests, here are a few things to check:
- Make sure you’re using a recent version of Python (3.6+).
- If you’re using pip, make sure you’ve upgraded to the latest version with
python -m pip install --upgrade pip
. - If you’re using conda, make sure your Anaconda distribution is up-to-date.
- Check that you have permissions to install packages on your system. You may need to use
sudo
or run your command shell as Administrator.
If you’re still having trouble, consult the requests documentation or ask for help on the requests GitHub issues page.
Usage Examples
With requests installed, let’s look at a couple of basic usage examples.
Making a Request
Let’s make a basic GET request to the GitHub API:
import requests
response = requests.get('https://api.github.com')
print(response.status_code)
# 200
print(response.text)
# '{"current_user_url":"https://api.github.com/user","authorizations_url":"https://api.github.com/authorizations", ...}'
We can see the response status code is 200, indicating the request was successful. The response text contains the JSON data returned by the API.
Web Scraping
requests is commonly used for web scraping. Let’s try scraping the HTML from a Wikipedia page:
import requests
url = 'https://en.wikipedia.org/wiki/Web_scraping'
response = requests.get(url)
print(response.status_code)
# 200
print(response.text)
# <!DOCTYPE html>
# <html class="client-nojs" lang="en" dir="ltr">
# <head>
# <meta charset="UTF-8"/>
# <title>Web scraping - Wikipedia</title>
# ...
# </body>
# </html>
We make a GET request to the Wikipedia article on web scraping and print out the complete HTML content of the page by accessing response.text
. You can then use a library like BeautifulSoup to parse and extract information from this HTML.
For more advanced web scraping needs, consider using a dedicated scraping service like Firecrawl. Firecrawl handles the complexities of web scraping, including proxy rotation, JavaScript rendering, and avoiding detection, so you can focus on working with the data. Check out the Python SDK here.
References
- Requests documentation: https://requests.readthedocs.io/
- Requests GitHub repository: https://github.com/psf/requests
- BeautifulSoup documentation: https://www.crummy.com/software/BeautifulSoup/bs4/doc/
- Firecrawl web scraping service: https://firecrawl.dev/
Ready to Build?
Start scraping web data for your AI apps today.
No credit card needed.
About the Author
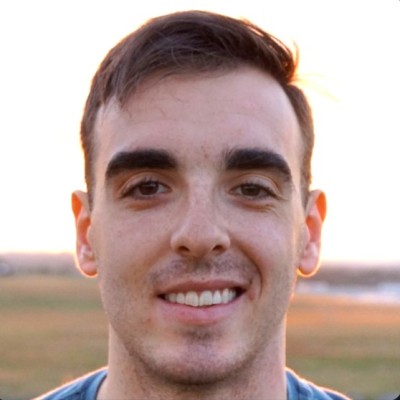
Eric Ciarla is the Chief Operating Officer (COO) of Firecrawl and leads marketing. He also worked on Mendable.ai and sold it to companies like Snapchat, Coinbase, and MongoDB. Previously worked at Ford and Fracta as a Data Scientist. Eric also co-founded SideGuide, a tool for learning code within VS Code with 50,000 users.
More articles by Eric Ciarla
Cloudflare Error 1015: How to solve it?
Cloudflare Error 1015 is a rate limiting error that occurs when Cloudflare detects that you are exceeding the request limit set by the website owner.
Build an agent that checks for website contradictions
Using Firecrawl and Claude to scrape your website's data and look for contradictions.
Getting Started with OpenAI's Predicted Outputs for Faster LLM Responses
A guide to leveraging Predicted Outputs to speed up LLM tasks with GPT-4o models.
How to easily install requests with pip and python
A tutorial on installing the requests library in Python using various methods, with usage examples and troubleshooting tips
How to quickly install BeautifulSoup with Python
A guide on installing the BeautifulSoup library in Python using various methods, with usage examples and troubleshooting tips
How to Use OpenAI's o1 Reasoning Models in Your Applications
Learn how to harness OpenAI's latest o1 series models for complex reasoning tasks in your apps.
Introducing Fire Engine for Firecrawl
The most scalable, reliable, and fast way to get web data for Firecrawl.
Firecrawl July 2024 Updates
Discover the latest features, integrations, and improvements in Firecrawl for July 2024.