How to quickly install BeautifulSoup with Python
BeautifulSoup is a Python library for pulling data out of HTML and XML files. It provides simple methods for navigating, searching, and modifying the parse tree, saving you hours of work. Beautiful Soup is great for web scraping projects where you need to extract specific pieces of information from web pages.
Some common use cases for BeautifulSoup include extracting article text or metadata from news sites, scraping product details and pricing from e-commerce stores, gathering data for machine learning datasets, and more.
In this tutorial, we’ll walk through several ways to get BeautifulSoup installed on your system and show you some basic usage examples to get started.
Installing BeautifulSoup
There are a few different ways you can install BeautifulSoup depending on your Python environment and preferences.
Using pip
The recommended way to install BeautifulSoup is with pip:
python -m pip install beautifulsoup4
This will install the latest version of BeautifulSoup 4. Make sure you have a recent version of Python (3.6+) and pip.
Using conda
If you’re using the Anaconda Python distribution, you can install BeautifulSoup from the conda-forge channel:
conda install -c conda-forge beautifulsoup4
In a virtual environment
It’s good practice to install Python packages in an isolated virtual environment for each project. You can set up BeautifulSoup in a new virtual environment like this:
python -m venv bsenv
source bsenv/bin/activate # On Windows, use `bsenv\Scripts\activate`
pip install beautifulsoup4
Troubleshooting
Here are a few things to check if you run into issues installing BeautifulSoup:
- Make sure your Python version is 3.6 or higher
- Upgrade pip to the latest version:
python -m pip install --upgrade pip
- If using conda, ensure your Anaconda installation is up-to-date
- Verify you have proper permissions to install packages. Use
sudo
or run the command prompt as an administrator if needed.
Check the BeautifulSoup documentation or post on Stack Overflow if you need further assistance.
Usage Examples
Let’s look at a couple quick examples of how to use BeautifulSoup once you have it installed.
Parsing HTML
Here’s how you can use BeautifulSoup to parse HTML retrieved from a web page:
from bs4 import BeautifulSoup
import requests
url = "https://mendable.ai"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.title.text)
# 'Example Domain'
We use the requests library to fetch the HTML from a URL, then pass it to BeautifulSoup to parse. This allows us to navigate and search the HTML using methods like find()
and select()
.
Extracting Data
BeautifulSoup makes it easy to extract data buried deep within nested HTML tags. For example, to get all the links from a page:
links = soup.find_all('a')
for link in links:
print(link.get('href'))
# 'https://www.firecrawl.dev/'
The find_all()
method retrieves all <a>
tag elements. We can then iterate through them and access attributes like the href
URL using get()
.
By chaining together find()
and select()
methods, you can precisely target elements and attributes to scrape from the messiest of HTML pages. BeautifulSoup is an indispensable tool for any Python web scraping project.
For more advanced web scraping projects, consider using a dedicated scraping service like Firecrawl. Firecrawl takes care of the tedious parts of web scraping, like proxy rotation, JavaScript rendering, and avoiding detection, allowing you to focus your efforts on working with the data itself. Check out the Python SDK here.
References
- BeautifulSoup documentation: https://www.crummy.com/software/BeautifulSoup/bs4/doc/
- Real Python’s BeautifulSoup Tutorial: https://realpython.com/beautiful-soup-web-scraper-python/
- Firecrawl web scraping service: https://firecrawl.dev/
Ready to Build?
Start scraping web data for your AI apps today.
No credit card needed.
About the Author
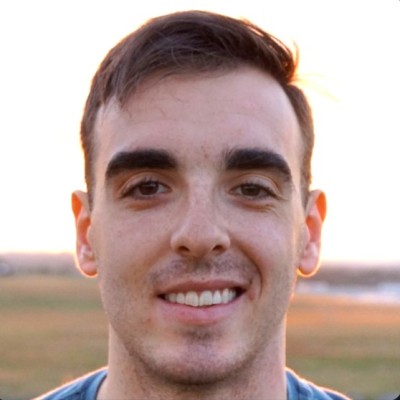
Eric Ciarla is the Chief Operating Officer (COO) of Firecrawl and leads marketing. He also worked on Mendable.ai and sold it to companies like Snapchat, Coinbase, and MongoDB. Previously worked at Ford and Fracta as a Data Scientist. Eric also co-founded SideGuide, a tool for learning code within VS Code with 50,000 users.
More articles by Eric Ciarla
Cloudflare Error 1015: How to solve it?
Cloudflare Error 1015 is a rate limiting error that occurs when Cloudflare detects that you are exceeding the request limit set by the website owner.
Build an agent that checks for website contradictions
Using Firecrawl and Claude to scrape your website's data and look for contradictions.
How to easily install requests with pip and python
A tutorial on installing the requests library in Python using various methods, with usage examples and troubleshooting tips
How to quickly install BeautifulSoup with Python
A guide on installing the BeautifulSoup library in Python using various methods, with usage examples and troubleshooting tips
How to Use OpenAI's o1 Reasoning Models in Your Applications
Learn how to harness OpenAI's latest o1 series models for complex reasoning tasks in your apps.
Introducing Fire Engine for Firecrawl
The most scalable, reliable, and fast way to get web data for Firecrawl.
Firecrawl July 2024 Updates
Discover the latest features, integrations, and improvements in Firecrawl for July 2024.
Launch Week I Recap
A look back at the new features and updates introduced during Firecrawl's inaugural Launch Week.