15 Python Web Scraping Projects: From Beginner to Advanced
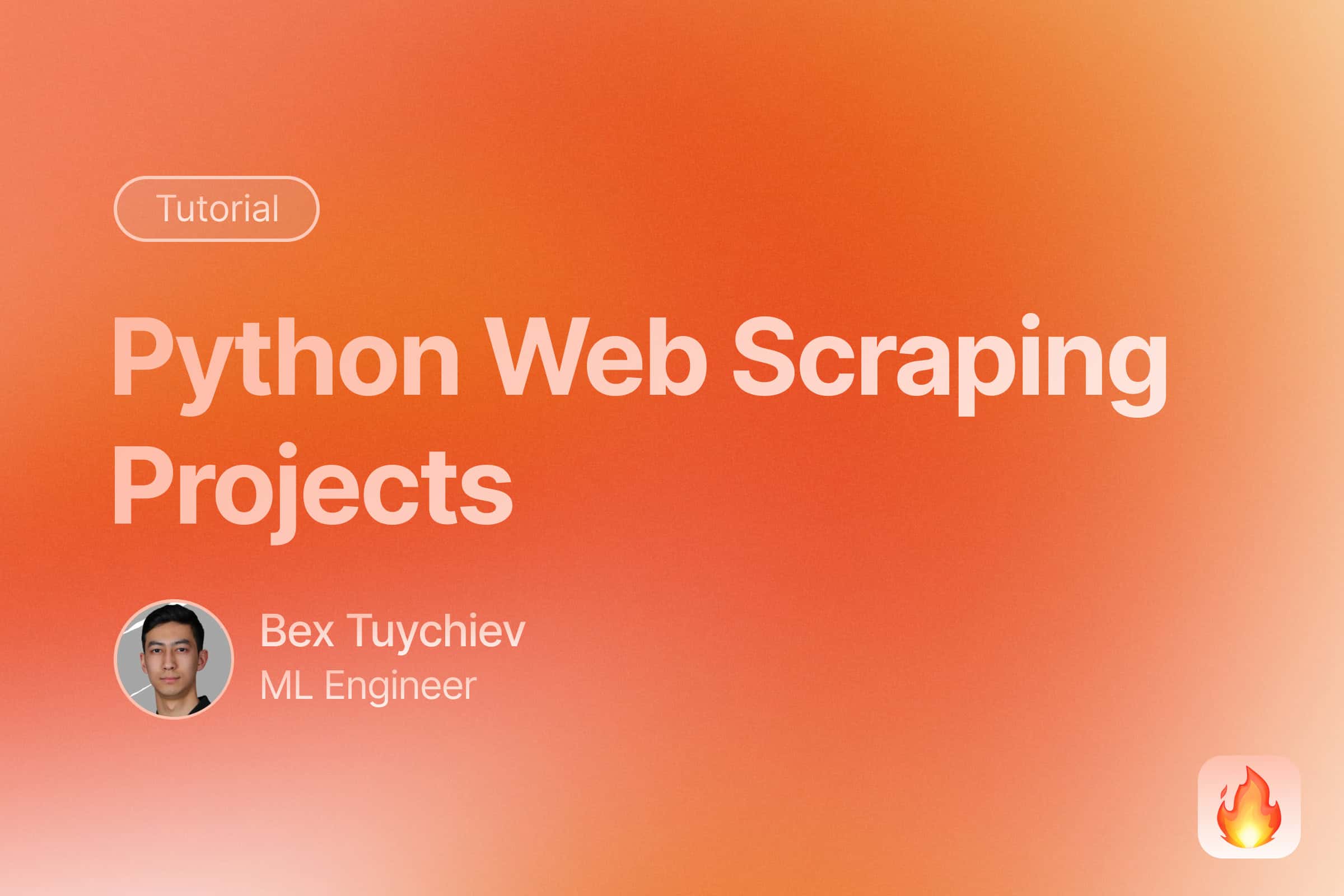
Introduction
Web scraping is one of the most powerful tools in a programmer’s arsenal, allowing you to gather data from across the internet automatically. It has countless applications like market research, competitive analysis, price monitoring, and data-driven decision making. The ability to extract structured data from web pages opens up endless possibilities for automation and analysis.
This guide outlines 15 web scraping project ideas in Python that progress from basic concepts to advanced techniques. Each project includes learning objectives, key technical concepts, and a structured development roadmap. While this guide doesn’t provide complete code implementations, it serves as a blueprint for your web scraping journey - helping you understand what to build and how to approach each challenge systematically.
Let’s begin by understanding the available tools and setting up our development environment. Then we’ll explore each project outline in detail, giving you a solid foundation to start building your own web scraping solutions.
Table of Contents
- Introduction
- Prerequisites
- Comparing Python Web Scraping Frameworks
- Setting Up Your Web Scraping Environment 5.Beginner Web Scraping Projects
- Intermediate Web Scraping Projects
- Advanced Web Scraping Projects
- Conclusion
Prerequisites
Before starting with these projects, you should have:
Required Skills
- Basic Python programming experience:
- Variables, data types, and operators
- Control structures (if/else, loops)
- Functions and basic error handling
- Working with lists and dictionaries
- Reading/writing files
- Installing and importing packages
- Basic web knowledge:
- Understanding of HTML structure
- Ability to use browser developer tools (inspect elements)
- Basic CSS selectors (class, id, tag selection)
- Understanding of URLs and query parameters
- Development environment:
- Python 3.x installed
- Ability to use command line/terminal
- Experience with pip package manager
- Text editor or IDE (VS Code, PyCharm, etc.)
Technical Requirements
- Computer with internet connection
- Modern web browser with developer tools
- Python 3.7+ installed
- Ability to install Python packages via pip
- Basic understanding of virtual environments
Optional but Helpful
- Understanding of:
- HTTP methods (GET, POST)
- JSON and CSV data formats
- Basic regular expressions
- Simple database concepts
- Git version control
- Experience with:
- pandas library for data manipulation
- Basic data visualization
- API interactions
- Web browser automation
Time Commitment
- 2-4 hours for setup and environment configuration
- 4-8 hours per beginner project
- Regular practice for skill improvement
If you’re new to web scraping, we recommend starting with the Weather Data Scraper or Recipe Collector projects, as they involve simpler website structures and basic data extraction patterns. The News Headlines Aggregator and Job Listing Monitor projects are more complex and might require additional learning about handling multiple data sources and pagination.
Comparing Python Web Scraping Frameworks For Your Projects
When starting with web scraping in Python, you’ll encounter several popular frameworks. Each has its strengths and ideal use cases. Let’s compare the main options to help you choose the right tool for your needs.
BeautifulSoup4
BeautifulSoup4 (BS4) is one of the most popular Python libraries for web scraping. It provides a simple and intuitive way to parse HTML and XML documents by creating a parse tree that can be navigated and searched. BS4 excels at extracting data from static web pages where JavaScript rendering isn’t required. The library works by transforming HTML code into a tree of Python objects, making it easy to locate and extract specific elements using methods like find()
and find_all()
. While it lacks some advanced features found in other frameworks, its simplicity and ease of use make it an excellent choice for beginners and straightforward scraping tasks.
Pros:
- Easy to learn and use
- Excellent documentation
- Great for parsing HTML/XML
- Lightweight and minimal dependencies
Cons:
- No JavaScript rendering
- Limited to basic HTML parsing
- No built-in download features
- Can be slow for large-scale scraping
Example usage:
from bs4 import BeautifulSoup
import requests
response = requests.get('https://example.com')
soup = BeautifulSoup(response.text, 'html.parser')
titles = soup.find_all('h1')
Selenium
Selenium is a powerful web automation framework that can control web browsers programmatically. Originally designed for web application testing, it has become a popular choice for web scraping, especially when dealing with dynamic websites that require JavaScript rendering. Selenium works by automating a real web browser, allowing it to interact with web pages just like a human user would - clicking buttons, filling forms, and handling dynamic content. This makes it particularly useful for scraping modern web applications where content is loaded dynamically through JavaScript.
Pros:
- Handles JavaScript-rendered content
- Supports browser automation
- Can interact with web elements
- Good for testing and scraping
Cons:
- Resource-intensive
- Slower than other solutions
- Requires browser drivers
- Complex setup and maintenance
Example Usage:
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get("https://example.com")
elements = driver.find_elements(By.CLASS_NAME, "product-title")
Scrapy
Scrapy is a comprehensive web scraping framework that provides a complete solution for extracting data from websites at scale. It’s designed as a fast, powerful, and extensible framework that can handle complex scraping tasks efficiently. Unlike simpler libraries, Scrapy provides a full suite of features including a crawling engine, data processing pipelines, and middleware components. It follows the principle of “batteries included” while remaining highly customizable for specific needs. Scrapy is particularly well-suited for large-scale scraping projects where performance and reliability are crucial.
Pros:
- High performance
- Built-in pipeline processing
- Extensive middleware support
- Robust error handling
Cons:
- Steep learning curve
- Complex configuration
- Limited JavaScript support
- Overkill for simple projects
Example Usage:
import scrapy
class ProductSpider(scrapy.Spider):
name = "products"
start_urls = ["https://example.com"]
def parse(self, response):
for product in response.css(".product"):
yield {
"name": product.css(".title::text").get(),
"price": product.css(".price::text").get(),
}
Firecrawl
Firecrawl represents a paradigm shift in web scraping by using AI to eliminate traditional scraping bottlenecks. Unlike conventional frameworks that require manual selector maintenance, Firecrawl uses natural language understanding to automatically identify and extract HTML element content based on semantic descriptions. This approach directly addresses the primary challenges faced in the projects outlined in this guide:
-
Development speed
- Traditional approach: Writing selectors, handling JavaScript, managing anti-bot measures (~2-3 days per site)
- Firecrawl approach: Define data schema, let AI handle extraction (~30 minutes per site)
-
Maintenance requirements
- Traditional approach: Regular updates when sites change, selector fixes, anti-bot adaptations
- Firecrawl approach: Schema remains stable, AI adapts to site changes automatically
-
Project implementation
- For the e-commerce projects: Built-in handling of dynamic pricing, AJAX requests, and anti-bot measures
- For news aggregation: Automatic content classification and extraction across different layouts
- For market research: Seamless handling of multiple site structures and authentication flows
Pros:
- AI-powered content extraction eliminates selector maintenance
- Automatic handling of JavaScript-rendered content
- Built-in anti-bot measures with enterprise-grade reliability
- Multiple output formats (JSON, CSV, structured objects)
- Site change resilience through semantic understanding
- Consistent extraction across different page layouts
Cons:
- Paid service (consider ROI vs. development time)
- API-dependent architecture
- Less granular control over parsing process
- May be overkill for simple, static sites
- Slower for large-scale operations
Example Implementation:
from firecrawl import FirecrawlApp
from pydantic import BaseModel, Field
class Product(BaseModel):
name: str = Field(description="The product name and title")
price: float = Field(description="The current price in USD")
description: str = Field(description="The product description text")
rating: float = Field(description="The average customer rating out of 5 stars")
num_reviews: int = Field(description="The total number of customer reviews")
availability: str = Field(description="The current availability status")
brand: str = Field(description="The product manufacturer or brand")
category: str = Field(description="The product category or department")
asin: str = Field(description="The Amazon Standard Identification Number")
app = FirecrawlApp()
data = app.scrape_url(
'https://www.amazon.com/gp/product/1718501900', # A sample Amazon product
params={
"formats": ['extract'],
"extract": {
"schema": Product.model_json_schema()
}
}
)
This example demonstrates how Firecrawl reduces complex e-commerce scraping to a simple schema definition. The same approach applies to all projects in this guide, potentially reducing development time from weeks to days. For production environments where reliability and maintenance efficiency are crucial, this automated approach often proves more cost-effective than maintaining custom scraping infrastructure.
Here is a table summarizing the differences between these tools:
Tool | Best For | Learning Curve | Key Features |
---|---|---|---|
BeautifulSoup4 | Static websites, Beginners | Easy | Simple API, Great documentation |
Selenium | Dynamic websites, Browser automation | Moderate | Full browser control, JavaScript support |
Scrapy | Large-scale projects | Steep | High performance, Extensive features |
Firecrawl | Production use, AI-powered scraping | Easy | Low maintenance, Built-in anti-bot |
Useful Resources:
- BeautifulSoup4 documentation
- Selenium documentation
- Scrapy documentation
- Firecrawl documentation
- Introduction to web scraping in Python tutorial
With these tools and resources at your disposal, you’re ready to start exploring web scraping in Python. Let’s move on to setting up your environment.
Setting Up Your Web Scraping Environment
Before diving into the projects, let’s set up our Python environment with the necessary tools and libraries. We’ll create a virtual environment and install the required packages.
- Create and activate a virtual environment
# Create a new virtual environment
python -m venv scraping-env
# Activate virtual environment
# On Windows:
scraping-env\Scripts\activate
# On macOS/Linux:
source scraping-env/bin/activate
- Install Required Packages
pip install requests beautifulsoup4 selenium scrapy firecrawl-py pandas
- Additional Setup for Selenium
If you plan to use Selenium, you’ll need to install a webdriver. For Chrome:
pip install webdriver-manager
- Basic Project Structure
Create a basic project structure to organize your code:
mkdir web_scraping_projects
cd web_scraping_projects
touch requirements.txt
Add the dependencies to requirements.txt
:
requests>=2.31.0
beautifulsoup4>=4.12.2
selenium>=4.15.2
scrapy>=2.11.0
firecrawl-py>=0.1.0
pandas>=2.1.3
webdriver-manager>=4.0.1
- Important Notes
- Always check a website’s robots.txt file before scraping
- Implement proper delays between requests (rate limiting)
- Consider using a user agent string to identify your scraper
- Handle errors and exceptions appropriately
- Store your API keys and sensitive data in environment variables
With this environment set up, you’ll be ready to tackle any of the projects in this tutorial, from beginner to advanced level. Each project may require additional specific setup steps, which will be covered in their respective sections.
Beginner Web Scraping Projects
Let’s start with some beginner-friendly web scraping projects that will help you build foundational skills.
1. Weather Data Scraper
A real-time weather data scraper for weather.com extracts temperature, humidity, wind speed and precipitation forecasts. The project serves as an introduction to fundamental web scraping concepts including HTTP requests, HTML parsing, and error handling.
This beginner-friendly project demonstrates proper web scraping practices through practical application, with opportunities to expand into historical trend analysis and multi-location comparisons. The core focus is on DOM navigation, rate limiting implementation, and efficient data storage techniques.
Learning objectives:
- Understanding HTML structure and basic DOM elements
- Making HTTP requests
- Parsing simple HTML responses
- Handling basic error cases
Proposed project steps:
-
Set up your development environment:
- Install required libraries (requests, beautifulsoup4)
- Create a new Python script file
- Configure your IDE/editor
-
Analyze the weather website structure:
- Open browser developer tools (F12)
- Inspect HTML elements for weather data
- Document CSS selectors for key elements
- Check robots.txt for scraping permissions
-
Build the basic scraper structure:
- Create a WeatherScraper class
- Add methods for making HTTP requests
- Implement user agent rotation
- Add request delay functionality
-
Implement data extraction:
- Write methods to parse temperature
- Extract humidity percentage
- Get wind speed and direction
- Collect precipitation forecast
- Parse “feels like” temperature
- Get weather condition description
-
Add error handling and validation:
- Implement request timeout handling
- Add retry logic for failed requests
- Validate extracted data types
- Handle missing data scenarios
- Log errors and exceptions
-
Create data storage functionality:
- Design CSV file structure
- Implement data cleaning
- Add timestamp to records
- Create append vs overwrite options
- Include location information
-
Test and refine:
- Test with multiple locations
- Verify data accuracy
- Optimize request patterns
- Add data validation checks
- Document known limitations
Key concepts to learn:
- HTTP requests and responses
- HTML parsing basics
- CSS selectors and HTML class/id attributes
- Data extraction patterns
- Basic error handling
Website suggestions:
- weather.com - Main weather data source with comprehensive information
- accuweather.com - Alternative source with detailed forecasts
- weatherunderground.com - Community-driven weather data
- openweathermap.org - Free API available for learning
- forecast.weather.gov - Official US weather data source
2. News Headlines Aggregator
A news headline aggregation system that pulls together breaking stories and trending content from multiple online news sources. The automated scraping engine visits major news websites on a schedule, extracting headlines, metadata, and key details into a unified data stream. The consolidated feed gives users a single interface to monitor news across publishers while handling the complexity of different site structures, update frequencies, and content formats behind the scenes.
Learning Objectives:
- Working with multiple data sources
- Handling different HTML structures
- Implementing proper delays between requests
- Basic data deduplication
Project steps:
-
Initial website selection and analysis
- Choose 2-3 news websites from suggested list
- Document each site’s robots.txt rules
- Identify optimal request intervals
- Map out common headline patterns
- Note any access restrictions
-
HTML structure analysis
- Inspect headline container elements
- Document headline text selectors
- Locate timestamp information
- Find article category/section tags
- Map author and source attribution
- Identify image thumbnail locations
-
Data model design
- Define headline object structure
- Create schema for metadata fields
- Plan timestamp standardization
- Design category classification
- Structure source tracking fields
- Add URL and unique ID fields
-
Individual scraper development
- Build base scraper class
- Implement site-specific extractors
- Add request delay handling
- Include user-agent rotation
- Set up error logging
- Add data validation checks
-
Data processing and storage
- Implement text cleaning
- Normalize timestamps
- Remove duplicate headlines
- Filter unwanted content
- Create CSV/JSON export
- Set up incremental updates
-
Integration and testing
- Combine multiple scrapers
- Add master scheduler
- Test with different intervals
- Validate combined output
- Monitor performance
- Document limitations
Key concepts to learn:
- Rate limiting and polite scraping
- Working with multiple websites
- Text normalization
- Basic data structures for aggregation
- Time handling in Python
Website suggestions:
- reuters.com - Major international news agency
- apnews.com - Associated Press news wire service
- bbc.com/news - International news coverage
- theguardian.com - Global news with good HTML structure
- aljazeera.com - International perspective on news
3. Book Price Tracker
Develop an automated price monitoring system that continuously scans multiple online bookstores to track price fluctuations for specific books. The tool will maintain a watchlist of titles, periodically check their current prices, and notify users when prices drop below certain thresholds or when significant discounts become available. This enables book enthusiasts to make cost-effective purchasing decisions by capitalizing on temporary price reductions across different retailers.
Learning objectives:
- Persistent data storage
- Price extraction and normalization
- Basic automation concepts
- Simple alert systems
Project steps:
- Analyze target bookstores
- Research and select online bookstores to monitor
- Study website structures and price display patterns
- Document required headers and request parameters
- Test rate limits and access restrictions
- Design data storage
- Create database tables for books and price history
- Define schema for watchlists and price thresholds
- Plan price tracking and comparison logic
- Set up automated backups
- Build price extraction system
- Implement separate scrapers for each bookstore
- Extract prices, availability and seller info
- Handle different currencies and formats
- Add error handling and retries
- Validate extracted data
- Implement automation
- Set up scheduled price checks
- Configure appropriate delays between requests
- Track successful/failed checks
- Implement retry logic for failures
- Monitor system performance
- Add notification system
- Create price threshold triggers
- Set up email notifications
- Add price drop alerts
- Generate price history reports
- Allow customizable alert preferences
Key concepts to learn:
- Database basics (SQLite or similar)
- Regular expressions for price extraction
- Scheduling with Python
- Email notifications
- Data comparison logic
Website suggestions:
- amazon.com - Large selection and dynamic pricing
- bookdepository.com - International book retailer
- barnesandnoble.com - Major US book retailer
- abebooks.com - Used and rare books marketplace
- bookfinder.com - Book price comparison site
4. Recipe Collector
Build an automated recipe scraping tool that collects detailed cooking information from food websites. The system will extract comprehensive recipe data including ingredient lists with measurements, step-by-step preparation instructions, cooking durations, serving sizes, and nutritional facts. This tool enables home cooks to easily aggregate and organize recipes from multiple sources into a standardized format.
Learning objectives:
- Handling nested HTML structures
- Extracting structured data
- Text cleaning and normalization
- Working with lists and complex data types
Project steps:
-
Analyze recipe website structures
- Study HTML structure of target recipe sites
- Identify common patterns for recipe components
- Document CSS selectors and XPaths for key elements
- Map variations between different sites
-
Design a recipe data model
- Create database schema for recipes
- Define fields for ingredients, instructions, metadata
- Plan data types and relationships
- Add support for images and rich media
- Include tags and categories
-
Implement extraction logic for recipe components
- Build scrapers for each target website
- Extract recipe title and description
- Parse ingredient lists with quantities and units
- Capture step-by-step instructions
- Get cooking times and temperatures
- Collect serving size information
- Extract nutritional data
- Download recipe images
-
Clean and normalize extracted data
- Standardize ingredient measurements
- Convert temperature units
- Normalize cooking durations
- Clean up formatting and special characters
- Handle missing or incomplete data
- Validate data consistency
- Remove duplicate recipes
-
Store recipes in a structured format
- Save to SQL/NoSQL database
- Export options to JSON/YAML
- Generate printable recipe cards
- Add search and filtering capabilities
- Implement recipe categorization
- Create backup system
Key concepts to learn:
- Complex HTML navigation
- Data cleaning techniques
- JSON/YAML data formats
- Nested data structures
- Text processing
Website suggestions:
- allrecipes.com - Large recipe database
- foodnetwork.com - Professional recipes
- epicurious.com - Curated recipe collection
- simplyrecipes.com - Well-structured recipes
- food.com - User-submitted recipes
5. Job Listing Monitor
Create an automated job search monitoring tool that continuously scans multiple job listing websites for new positions matching user-defined criteria. The tool will track key details like job titles, companies, locations, salaries, and requirements. Users can specify search filters such as keywords, experience level, job type (remote/hybrid/onsite), and salary range. The system will store listings in a database and notify users of new matches via email or other alerts. This helps job seekers stay on top of opportunities without manually checking multiple sites.
The tool can integrate with major job boards like LinkedIn, Indeed, Glassdoor and company career pages. It will handle different site structures, login requirements, and listing formats while respecting rate limits and terms of service. Advanced features could include sentiment analysis of job descriptions, automatic resume submission, and tracking application status across multiple positions.
Learning objectives:
- Working with search parameters
- Handling pagination
- Form submission
- Data filtering
Project steps:
-
Set up initial project structure and dependencies
- Create virtual environment
- Install required libraries
- Set up database (SQLite/PostgreSQL)
- Configure logging and error handling
- Set up email notification system
-
Implement site-specific scrapers
- Analyze HTML structure of each job board
- Handle authentication if required
- Create separate scraper classes for each site (one is enough if you are using Firecrawl)
- Implement rate limiting and rotating user agents
- Add proxy support for avoiding IP blocks
- Handle JavaScript-rendered content with Selenium (no need if you are using Firecrawl)
-
Build search parameter system
- Create configuration for search criteria
- Implement URL parameter generation
- Handle different parameter formats per site
- Add validation for search inputs
- Support multiple search profiles
- Implement location-based searching
-
Develop listing extraction logic
- Extract job details (title, company, location, etc)
- Parse salary information
- Clean and standardize data format
- Handle missing/incomplete data
- Extract application requirements
- Identify remote/hybrid/onsite status
- Parse required skills and experience
-
Create storage and monitoring system
- Design database schema
- Implement data deduplication
- Track listing history/changes
- Set up automated monitoring schedule
- Create email alert templates
- Build basic web interface for results
- Add export functionality
Key concepts to learn:
- URL parameters and query strings
- HTML forms and POST requests
- Pagination handling
- Data filtering techniques
- Incremental data updates
Website suggestions:
- linkedin.com - Professional networking and job site
- indeed.com - Large job search engine
- glassdoor.com - Company reviews and job listings
- monster.com - Global job search platform
- dice.com - Technology job board
- careerbuilder.com - Major US job site
Intermediate Web Scraping Projects
These projects build upon basic scraping concepts and introduce more complex scenarios and techniques.
1. E-commerce Price Comparison Tool
Build a sophisticated price comparison system monitoring major e-commerce platforms like Amazon, eBay, Walmart and Best Buy. The tool tracks products via SKUs and model numbers, scraping pricing data at configurable intervals. It normalizes data by mapping equivalent items and standardizing prices, shipping costs, and seller information across platforms.
A dashboard interface displays historical price trends, sends price drop alerts via email/SMS, and recommends optimal purchase timing based on seasonal patterns and historical lows. The system handles JavaScript-rendered content, dynamic AJAX requests, and anti-bot measures while maintaining data in both SQL and NoSQL stores.
Key technical challenges include managing product variants, currency conversion, and adapting to frequent site layout changes while ensuring data accuracy and consistency.
Read our separate guide on building an Amazon price tracking application using Firecrawl for the basic version of this project.
Learning objectives:
- Multi-site data aggregation
- Price normalization techniques
- Advanced rate limiting
- Proxy rotation
- Database optimization
Project steps:
-
Design system architecture
- Plan database schema for products and prices
- Design API structure for data access
- Set up proxy management system
- Configure rate limiting rules
- Plan data update intervals
-
Implement core scraping functionality
- Create base scraper class
- Add proxy rotation mechanism
- Implement user agent rotation
- Set up request queuing
- Add retry logic
- Handle JavaScript rendering
- Configure session management
-
Build product matching system
- Implement product identification
- Create fuzzy matching algorithms
- Handle variant products
- Normalize product names
- Match product specifications
- Track product availability
-
Develop price analysis features
- Track historical prices
- Calculate price trends
- Identify price patterns
- Generate price alerts
- Create price prediction models
- Compare shipping costs
- Track discount patterns
-
Create reporting system
- Build price comparison reports
- Generate trend analysis
- Create price alert notifications
- Export data in multiple formats
- Schedule automated reports
- Track price history
Key concepts to learn:
- Advanced rate limiting
- Proxy management
- Product matching algorithms
- Price normalization
- Historical data tracking
Website suggestions:
- amazon.com - Large product database
- walmart.com - Major retailer
- bestbuy.com - Electronics focus
- target.com - Retail products
- newegg.com - Tech products
2. Social Media Analytics Tool
Build a comprehensive social media analytics platform that combines web scraping, API integration, and real-time monitoring capabilities. The system will aggregate engagement metrics and content across major social networks, process JavaScript-heavy pages, and provide actionable insights through customizable dashboards. Key features include sentiment analysis of comments, competitive benchmarking, and automated trend detection. The tool emphasizes scalable data collection while respecting rate limits and platform terms of service.
Learning objectives:
- JavaScript rendering
- API integration
- Real-time monitoring
- Data visualization
- Engagement metrics analysis
Project steps:
-
Platform analysis and setup
- Research API limitations
- Document scraping restrictions
- Set up authentication
- Plan data collection strategy
- Configure monitoring intervals
-
Implement data collection
- Create platform-specific scrapers
- Handle JavaScript rendering
- Implement API calls
- Track rate limits
- Monitor API quotas
- Handle pagination
- Collect media content
-
Build analytics engine
- Calculate engagement rates
- Track follower growth
- Analyze posting patterns
- Monitor hashtag performance
- Measure audience interaction
- Generate sentiment analysis
- Track competitor metrics
-
Develop visualization system
- Create interactive dashboards
- Generate trend graphs
- Build comparison charts
- Display real-time metrics
- Create export options
- Generate automated reports
-
Add monitoring features
- Set up real-time tracking
- Create alert system
- Monitor competitor activity
- Track brand mentions
- Generate periodic reports
- Implement custom metrics
Key concepts to learn:
- API integration
- Real-time data collection
- Engagement metrics
- Data visualization
- JavaScript handling
Website suggestions:
- twitter.com - Real-time social updates
- instagram.com - Visual content platform
- facebook.com - Social networking
- linkedin.com - Professional network
- reddit.com - Community discussions
3. Real Estate Market Analyzer
Develop a comprehensive real estate market analysis tool that collects and analyzes property listings from multiple sources. The system will track prices, property features, market trends, and neighborhood statistics to provide insights into real estate market conditions. This project focuses on handling pagination, geographic data, and large datasets.
Learning objectives:
- Geographic data handling
- Advanced pagination
- Data relationships
- Market analysis
- Database optimization
Project steps:
-
Set up data collection framework
- Design database schema
- Configure geocoding system
- Set up mapping integration
- Plan data update frequency
- Configure backup system
-
Implement listing collection
- Create site-specific scrapers
- Handle dynamic loading
- Process pagination
- Extract property details
- Collect images and media
- Parse property features
- Handle location data
-
Build analysis system
- Calculate market trends
- Analyze price per square foot
- Track inventory levels
- Monitor days on market
- Compare neighborhood stats
- Generate market reports
- Create price predictions
-
Develop visualization tools
- Create interactive maps
- Build trend graphs
- Display comparative analysis
- Show market indicators
- Generate heat maps
- Create property reports
-
Add advanced features
- Implement search filters
- Add custom alerts
- Create watchlists
- Generate market reports
- Track favorite properties
- Monitor price changes
Key concepts to learn:
- Geographic data processing
- Complex pagination
- Data relationships
- Market analysis
- Mapping integration
Website suggestions:
- zillow.com - Real estate listings
- realtor.com - Property database
- trulia.com - Housing market data
- redfin.com - Real estate platform
- homes.com - Property listings
4. Academic Research Aggregator
Create a comprehensive academic research aggregator that collects scholarly articles, papers, and publications from multiple academic databases and repositories. The system will track research papers, citations, author information, and publication metrics to help researchers stay updated with the latest developments in their field.
Learning objectives:
- PDF parsing and extraction
- Citation network analysis
- Academic API integration
- Complex search parameters
- Large dataset management
Project steps:
-
Source identification and setup
- Research academic databases
- Document API access requirements
- Set up authentication systems
- Plan data collection strategy
- Configure access protocols
- Handle rate limitations
-
Implement data collection
- Create database-specific scrapers
- Handle PDF downloads
- Extract paper metadata
- Parse citations
- Track author information
- Collect publication dates
- Handle multiple languages
-
Build citation analysis system
- Track citation networks
- Calculate impact factors
- Analyze author networks
- Monitor research trends
- Generate citation graphs
- Track paper influence
- Identify key papers
-
Develop search and filtering
- Implement advanced search
- Add field-specific filters
- Create topic clustering
- Enable author tracking
- Support boolean queries
- Add relevance ranking
- Enable export options
-
Create visualization and reporting
- Generate citation networks
- Create author collaboration maps
- Display research trends
- Show topic evolution
- Create custom reports
- Enable data export
Key concepts to learn:
- PDF text extraction
- Network analysis
- Academic APIs
- Complex search logic
- Large-scale data processing
Website suggestions:
- scholar.google.com - Academic search engine
- arxiv.org - Research paper repository
- sciencedirect.com - Scientific publications
- ieee.org - Technical papers
- pubmed.gov - Medical research
5. Financial Market Data Analyzer
Build a sophisticated financial market analysis tool that collects and processes data from multiple financial sources including stock markets, cryptocurrency exchanges, and forex platforms. The system will track prices, trading volumes, market indicators, and news sentiment to provide comprehensive market insights.
Learning objectives:
- Real-time data handling
- WebSocket connections
- Financial calculations
- Time series analysis
- News sentiment analysis
Project steps:
-
Data source integration
- Set up API connections
- Configure WebSocket feeds
- Implement rate limiting
- Handle authentication
- Manage data streams
- Plan backup sources
-
Market data collection
- Track price movements
- Monitor trading volume
- Calculate market indicators
- Record order book data
- Track market depth
- Handle multiple exchanges
- Process tick data
-
Build analysis engine
- Implement technical indicators
- Calculate market metrics
- Process trading signals
- Analyze price patterns
- Generate market alerts
- Track correlations
- Monitor volatility
-
Develop news analysis
- Collect financial news
- Process news sentiment
- Track market impact
- Monitor social media
- Analyze announcement effects
- Generate news alerts
-
Create visualization system
- Build price charts
- Display market indicators
- Show volume analysis
- Create correlation maps
- Generate trading signals
- Enable custom dashboards
Key concepts to learn:
- WebSocket programming
- Real-time data processing
- Financial calculations
- Market analysis
- News sentiment analysis
Website suggestions:
- finance.yahoo.com - Financial data
- marketwatch.com - Market news
- investing.com - Trading data
- tradingview.com - Technical analysis
- coinmarketcap.com - Crypto markets
Advanced Web Scraping Projects
These projects represent complex, production-grade applications that combine multiple advanced concepts and require sophisticated architecture decisions. They’re ideal for developers who have mastered basic and intermediate scraping techniques.
1. Multi-threaded News Aggregator
Build an enterprise-grade news aggregation system that uses concurrent processing to efficiently collect and analyze news from hundreds of sources simultaneously. The system will handle rate limiting, proxy rotation, and load balancing while maintaining high throughput and data accuracy. This project focuses on scalability and performance optimization.
Learning objectives:
- Concurrent programming
- Thread/Process management
- Queue systems
- Load balancing
- Performance optimization
Project steps:
-
Design concurrent architecture
- Plan threading strategy
- Design queue system
- Configure worker pools
- Set up load balancing
- Plan error handling
- Implement logging system
- Design monitoring tools
-
Build core scraping engine
- Create worker threads
- Implement task queue
- Set up proxy rotation
- Handle rate limiting
- Manage session pools
- Configure retries
- Monitor performance
-
Develop content processing
- Implement NLP analysis
- Extract key information
- Classify content
- Detect duplicates
- Process media content
- Handle multiple languages
- Generate summaries
-
Create storage and indexing
- Design database sharding
- Implement caching
- Set up search indexing
- Manage data retention
- Handle data validation
- Configure backups
- Optimize queries
-
Build monitoring system
- Track worker status
- Monitor queue health
- Measure throughput
- Track error rates
- Generate alerts
- Create dashboards
- Log performance metrics
Key concepts to learn:
- Thread synchronization
- Queue management
- Resource pooling
- Performance monitoring
- System optimization
Website suggestions:
- reuters.com - International news
- apnews.com - News wire service
- bloomberg.com - Financial news
- nytimes.com - News articles
- wsj.com - Business news
2. Distributed Web Archive System
Build a distributed web archiving system that preserves historical versions of websites across a network of nodes. The system will handle massive-scale crawling, content deduplication, versioning, and provide a searchable interface to access archived content. Think of it as building your own Internet Archive Wayback Machine with distributed architecture.
Learning objectives:
- Distributed systems architecture
- Content-addressable storage
- Version control concepts
- Distributed crawling
- Large-scale search
Project steps:
-
Design distributed architecture
- Plan node communication
- Design content addressing
- Configure storage sharding
- Implement consensus protocol
- Set up service discovery
- Plan failure recovery
- Design replication strategy
-
Build core archiving engine
- Implement snapshot system
- Handle resource capturing
- Process embedded content
- Manage asset dependencies
- Create versioning system
- Handle redirects
- Implement diff detection
-
Develop distributed crawler
- Create crawler nodes
- Implement work distribution
- Handle URL deduplication
- Manage crawl frontiers
- Process robots.txt
- Configure politeness rules
- Monitor node health
-
Create storage and indexing
- Implement content hashing
- Build merkle trees
- Create delta storage
- Set up distributed index
- Handle data replication
- Manage storage quotas
- Optimize retrieval
-
Build access interface
- Create temporal navigation
- Implement diff viewing
- Enable full-text search
- Build API endpoints
- Create admin dashboard
- Enable export options
- Handle access control
Key concepts to learn:
- Distributed systems
- Content addressing
- Merkle trees
- Consensus protocols
- Temporal data models
Technical requirements:
- Distributed database (e.g., Cassandra)
- Message queue system (e.g., Kafka)
- Search engine (e.g., Elasticsearch)
- Content-addressable storage
- Load balancers
- Service mesh
- Monitoring system
Advanced features:
- Temporal graph analysis
- Content change detection
- Link integrity verification
- Resource deduplication
- Distributed consensus
- Automated preservation
- Access control policies
This project combines distributed systems concepts with web archiving challenges, requiring deep understanding of both scalable architecture and content preservation techniques. It’s particularly relevant for organizations needing to maintain compliant records of web content or researchers studying web evolution patterns.
3. Automated Market Research Tool
Create a comprehensive market research platform that combines web scraping, data analysis, and automated reporting to provide competitive intelligence and market insights. The system will track competitors, analyze market trends, and generate detailed reports automatically.
Learning objectives:
- Large-scale data collection
- Advanced analytics
- Automated reporting
- Competitive analysis
- Market intelligence
Project steps:
-
Design research framework
- Define data sources
- Plan collection strategy
- Design analysis pipeline
- Configure reporting system
- Set up monitoring
- Plan data storage
- Configure backup systems
-
Implement data collection
- Create source scrapers
- Handle authentication
- Manage rate limits
- Process structured data
- Extract unstructured content
- Track changes
- Validate data quality
-
Build analysis engine
- Process market data
- Analyze trends
- Track competitors
- Generate insights
- Calculate metrics
- Identify patterns
- Create predictions
-
Develop reporting system
- Generate automated reports
- Create visualizations
- Build interactive dashboards
- Enable customization
- Schedule updates
- Handle distribution
- Track engagement
-
Add intelligence features
- Implement trend detection
- Create alerts system
- Enable custom analysis
- Build recommendation engine
- Generate insights
- Track KPIs
- Monitor competition
Key concepts to learn:
- Market analysis
- Report automation
- Data visualization
- Competitive intelligence
- Trend analysis
Website suggestions:
- Company websites
- Industry news sites
- Government databases
- Social media platforms
- Review sites
4. Competitive Intelligence Dashboard
Build a real-time competitive intelligence platform that monitors competitor activities across multiple channels including websites, social media, and news sources. The system will provide automated alerts and analysis of competitive movements in the market.
Learning objectives:
- Real-time monitoring
- Complex automation
- Data warehousing
- Dashboard development
- Alert systems
Project steps:
-
Set up monitoring system
- Configure data sources
- Set up real-time tracking
- Implement change detection
- Design alert system
- Plan data storage
- Configure monitoring rules
- Handle authentication
-
Build data collection
- Create source scrapers
- Handle dynamic content
- Process structured data
- Extract unstructured content
- Track changes
- Monitor social media
- Collect news mentions
-
Develop analysis engine
- Process competitor data
- Analyze market position
- Track product changes
- Monitor pricing
- Analyze marketing
- Track customer sentiment
- Generate insights
-
Create dashboard interface
- Build real-time displays
- Create interactive charts
- Enable custom views
- Implement filtering
- Add search functionality
- Enable data export
- Configure alerts
-
Implement alert system
- Set up notification rules
- Create custom triggers
- Handle priority levels
- Enable user preferences
- Track alert history
- Generate summaries
- Monitor effectiveness
Key concepts to learn:
- Real-time monitoring
- Change detection
- Alert systems
- Dashboard design
- Competitive analysis
Website suggestions:
- Competitor websites
- Social media platforms
- News aggregators
- Review sites
- Industry forums
5. Full-Stack Scraping Platform
Develop a complete web scraping platform with a user interface that allows non-technical users to create and manage scraping tasks. The system will include visual scraping tools, scheduling, monitoring, and data export capabilities.
Learning objectives:
- Full-stack development
- API design
- Frontend development
- System architecture
- User management
Project steps:
-
Design system architecture
- Plan component structure
- Design API endpoints
- Configure databases
- Set up authentication
- Plan scaling strategy
- Design monitoring
- Configure deployment
-
Build backend system
- Create API endpoints
- Implement authentication
- Handle task management
- Process scheduling
- Manage user data
- Handle file storage
- Configure security
-
Develop scraping engine
- Create scraper framework
- Handle different sites
- Manage sessions
- Process rate limits
- Handle errors
- Validate data
- Monitor performance
-
Create frontend interface
- Build user dashboard
- Create task manager
- Implement scheduling
- Show monitoring data
- Enable configuration
- Handle data export
- Display results
-
Add advanced features
- Visual scraper builder
- Template system
- Export options
- Notification system
- User management
- Usage analytics
- API documentation
Key concepts to learn:
- System architecture
- API development
- Frontend frameworks
- User management
- Deployment
Website suggestions:
- Any website (platform should be generic)
- Test sites for development
- Documentation resources
- API references
- Example targets
Conclusion
Web scraping is a powerful skill that opens up endless possibilities for data collection and analysis. Through these 15 projects, ranging from basic weather scrapers to advanced AI-powered content extraction systems, you’ve seen how web scraping can be applied to solve real-world problems across different domains.
Key takeaways from these projects include:
- Start with simpler projects to build foundational skills
- Progress gradually to more complex architectures
- Focus on ethical scraping practices and website policies
- Use appropriate tools based on project requirements
- Implement proper error handling and data validation
- Consider scalability and maintenance from the start
Whether you’re building a simple price tracker or a full-scale market intelligence platform, the principles and techniques covered in these projects will serve as a solid foundation for your web scraping journey. Remember to always check robots.txt files, implement appropriate delays, and respect website terms of service while scraping.
For your next steps, pick a project that aligns with your current skill level and start building. The best way to learn web scraping is through hands-on practice and real-world applications. As you gain confidence, gradually tackle more complex projects and keep exploring new tools and techniques in this ever-evolving field.
On this page
Introduction
Table of Contents
Prerequisites
Required Skills
Technical Requirements
Optional but Helpful
Time Commitment
Comparing Python Web Scraping Frameworks For Your Projects
BeautifulSoup4
Selenium
Scrapy
Firecrawl
Setting Up Your Web Scraping Environment
Beginner Web Scraping Projects
1. Weather Data Scraper
2. News Headlines Aggregator
3. Book Price Tracker
4. Recipe Collector
5. Job Listing Monitor
Intermediate Web Scraping Projects
1. E-commerce Price Comparison Tool
2. Social Media Analytics Tool
3. Real Estate Market Analyzer
4. Academic Research Aggregator
5. Financial Market Data Analyzer
Advanced Web Scraping Projects
1. Multi-threaded News Aggregator
2. Distributed Web Archive System
3. Automated Market Research Tool
4. Competitive Intelligence Dashboard
5. Full-Stack Scraping Platform
Conclusion
About the Author
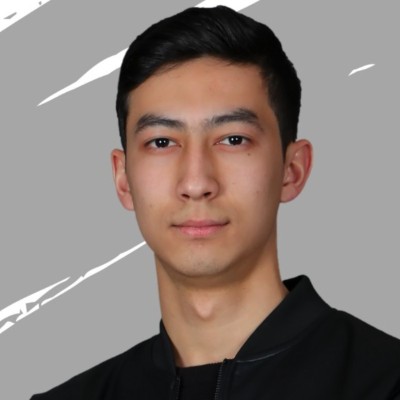
Bex is a Top 10 AI writer on Medium and a Kaggle Master with over 15k followers. He loves writing detailed guides, tutorials, and notebooks on complex data science and machine learning topics
More articles by Bex Tuychiev
Top 7 AI-Powered Web Scraping Solutions in 2025
Discover the most advanced AI web scraping tools that are revolutionizing data extraction with natural language processing and machine learning capabilities.
Building an Automated Price Tracking Tool
Learn how to build an automated price tracker in Python that monitors e-commerce prices and sends alerts when prices drop.
Web Scraping Automation: How to Run Scrapers on a Schedule
Learn how to automate web scraping in Python using free scheduling tools to run scrapers reliably in 2025.
Automated Data Collection - A Comprehensive Guide
A comprehensive guide to building robust automated data collection systems using modern tools and best practices.
Top 9 Browser Automation Tools for Web Testing and Scraping in 2025
Comprehensive comparison of the best browser automation frameworks including Selenium, Playwright, Puppeteer, and Cypress for web testing, data extraction, and workflow automation with implementation guides.
BeautifulSoup4 vs. Scrapy - A Comprehensive Comparison for Web Scraping in Python
A comprehensive comparison of BeautifulSoup4 and Scrapy to help you choose the right Python web scraping tool.
How to Build a Client Relationship Tree Visualization Tool in Python
Build an application that discovers and visualizes client relationships by scraping websites with Firecrawl and presenting the data in an interactive tree structure using Streamlit and PyVis.
How to Build an Automated Competitor Price Monitoring System with Python
Learn how to build an automated price monitoring system in Python to track and compare competitor prices across e-commerce sites.